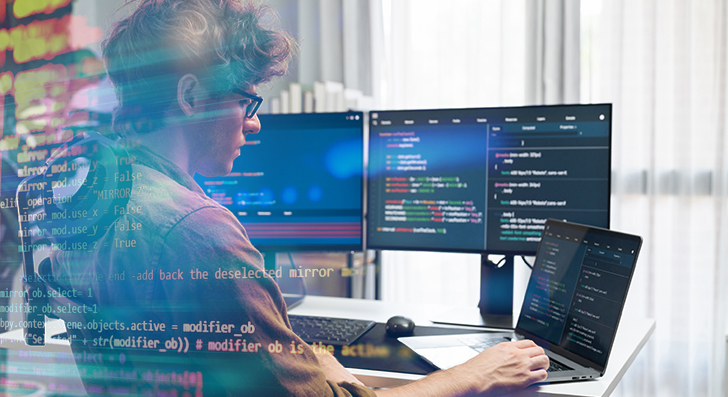
Scalability indicates your software can manage growth—extra people, far more info, and even more visitors—without breaking. Being a developer, building with scalability in your mind saves time and worry later on. Right here’s a transparent and functional manual that will help you get started by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is just not a thing you bolt on later on—it ought to be portion of one's system from the beginning. Many apps fail if they develop rapid simply because the first style can’t take care of the additional load. Like a developer, you might want to Feel early regarding how your system will behave stressed.
Start by creating your architecture for being adaptable. Steer clear of monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial elements. Just about every module or service can scale on its own with no influencing The complete method.
Also, think of your databases from day a single. Will it will need to take care of a million customers or perhaps a hundred? Select the suitable style—relational or NoSQL—determined by how your facts will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
A different vital point is to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day disorders. Think about what would happen if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that guidance scaling, like concept queues or function-driven techniques. These assist your app handle a lot more requests without having obtaining overloaded.
Whenever you build with scalability in your mind, you are not just getting ready for success—you're reducing long term headaches. A perfectly-prepared program is easier to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Databases
Picking out the proper database is usually a essential Portion of building scalable purposes. Not all databases are created the identical, and using the wrong you can slow you down or simply lead to failures as your app grows.
Start out by comprehension your information. Can it be very structured, like rows within a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and regularity. In addition they assist scaling strategies like read replicas, indexing, and partitioning to manage more targeted traffic and information.
If the info is a lot more flexible—like consumer exercise logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your examine and publish styles. Are you currently undertaking lots of reads with fewer writes? Use caching and browse replicas. Will you be handling a hefty publish load? Take a look at databases that could tackle higher compose throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel in advance. You might not need Superior scaling characteristics now, but picking a databases that supports them usually means you won’t need to switch later.
Use indexing to speed up queries. Prevent unwanted joins. Normalize or denormalize your details depending on your access styles. And always monitor database performance as you grow.
In short, the proper database depends upon your app’s structure, velocity requires, And exactly how you be expecting it to improve. Just take time to choose properly—it’ll help save a great deal of difficulties later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Inadequately penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial to build efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove anything at all pointless. Don’t pick the most intricate Remedy if a straightforward just one operates. Keep your features brief, concentrated, and simple to test. Use profiling tools to search out bottlenecks—areas where your code can take as well extensive to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These frequently gradual issues down in excess of the code itself. Ensure that Each and every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and instead find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Specifically throughout large tables.
Should you see exactly the same knowledge remaining asked for many times, use caching. Shop the outcome quickly using resources like Redis or Memcached therefore you don’t have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to take a look at with significant datasets. Code and queries that work good with 100 information may possibly crash if they have to handle 1 million.
In brief, scalable apps are quickly apps. Keep your code restricted, your queries lean, and use caching when necessary. These methods support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of additional buyers plus more traffic. If every thing goes via 1 server, it'll quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these applications assistance maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. As an alternative to a single server carrying out all of the work, the load balancer routes buyers to unique servers based on availability. This suggests no solitary server gets overloaded. If a person server goes more info down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused swiftly. When end users request a similar data once more—like an item webpage or a profile—you don’t should fetch it from your databases each and every time. You can provide it from the cache.
There's two typical kinds of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Consumer-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching cuts down database load, increases pace, and will make your app additional efficient.
Use caching for things which don’t improve usually. And normally ensure your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are straightforward but highly effective tools. Collectively, they help your app cope with more consumers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that allow your app improve conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t should purchase hardware or guess potential capability. When site visitors will increase, it is possible to insert extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection applications. You could deal with making your application as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every thing it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application makes use of numerous containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular element within your app crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In short, employing cloud and container tools suggests you are able to scale rapid, deploy effortlessly, and Get better rapidly when problems come about. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, cut down threat, and make it easier to stay focused on constructing, not correcting.
Keep track of Almost everything
For those who don’t keep track of your software, you received’t know when items go Erroneous. Checking helps you see how your app is undertaking, location issues early, and make far better selections as your application grows. It’s a vital part of developing scalable programs.
Start out by monitoring essential metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and companies are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just watch your servers—observe your application much too. Regulate how much time it takes for users to load pages, how frequently faults happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. For instance, In case your response time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This assists you repair issues speedy, generally in advance of consumers even recognize.
Monitoring is usually handy once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you may roll it again ahead of it leads to serious problems.
As your app grows, targeted visitors and knowledge boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the correct tools in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not just about recognizing failures—it’s about comprehending your system and making certain it really works effectively, even stressed.
Last Views
Scalability isn’t just for massive companies. Even modest applications want a solid foundation. By planning carefully, optimizing properly, and utilizing the right equipment, you can Construct applications that grow easily devoid of breaking under pressure. Commence smaller, think massive, and Establish wise.